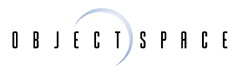
| JGL - The Generic Collection Library for Java |
All Packages Class Hierarchy This Package Previous Next Index
Class com.objectspace.jgl.util.Randomizer
java.lang.Object
|
+----java.util.Random
|
+----com.objectspace.jgl.util.Randomizer
- public class Randomizer
- extends Random
An easy-to-use random number generator.
-
Randomizer()
- Initializes the generator using a seed based on the current time.
-
Randomizer(long)
- Initializes the generator using a specific seed; useful for generating
a repeatable stream of random numbers.
-
getDouble(double)
- Generate a random number using the default generator.
-
getDouble(double, double)
- Generate a random number using the default generator.
-
getFloat(float)
- Generate a random number using the default generator.
-
getFloat(float, float)
- Generate a random number using the default generator.
-
getInt(int)
- Generate a random number using the default generator.
-
getInt(int, int)
- Generate a random number using the default generator.
-
getLong(long)
- Generate a random number using the default generator.
-
getLong(long, long)
- Generate a random number using the default generator.
-
nextDouble(double)
- Generates a double value between 1.0 and the given limit.
-
nextDouble(double, double)
- Generates a double value between the given limits.
-
nextFloat(float)
- Generates a float value between 1.0 and the given limit.
-
nextFloat(float, float)
- Generates a float value between the given limits.
-
nextInt(int)
- Generates an int value between 1 and the given limit.
-
nextInt(int, int)
- Generates an int value between the given limits.
-
nextLong(long)
- Generates a long value between 1 and the given limit.
-
nextLong(long, long)
- Generates a long value between the given limits.
Randomizer
public Randomizer()
- Initializes the generator using a seed based on the current time.
Randomizer
public Randomizer(long seed)
- Initializes the generator using a specific seed; useful for generating
a repeatable stream of random numbers.
- Parameters:
- seed - The initial seed.
- See Also:
- setSeed
nextInt
public int nextInt(int hi)
- Generates an int value between 1 and the given limit.
- Parameters:
- hi - The upper bound.
- Returns:
- An integer value.
- See Also:
- nextInt
nextInt
public int nextInt(int lo,
int hi)
- Generates an int value between the given limits.
- Parameters:
- lo - The lower bound.
- hi - The upper bound.
- Returns:
- An integer value.
- See Also:
- nextInt
nextLong
public long nextLong(long hi)
- Generates a long value between 1 and the given limit.
- Parameters:
- hi - The upper bound.
- Returns:
- A long value.
- See Also:
- nextLong
nextLong
public long nextLong(long lo,
long hi)
- Generates a long value between the given limits.
- Parameters:
- lo - The lower bound.
- hi - The upper bound.
- Returns:
- A long integer value.
- See Also:
- nextLong
nextFloat
public float nextFloat(float hi)
- Generates a float value between 1.0 and the given limit.
- Parameters:
- hi - The upper bound.
- Returns:
- A float value.
- See Also:
- nextFloat
nextFloat
public float nextFloat(float lo,
float hi)
- Generates a float value between the given limits.
- Parameters:
- lo - The lower bound.
- hi - The upper bound.
- Returns:
- A float value.
- See Also:
- nextFloat
nextDouble
public double nextDouble(double hi)
- Generates a double value between 1.0 and the given limit.
- Parameters:
- hi - The upper bound.
- Returns:
- A double value.
- See Also:
- nextDouble
nextDouble
public double nextDouble(double lo,
double hi)
- Generates a double value between the given limits.
- Parameters:
- lo - The lower bound.
- hi - The upper bound.
- Returns:
- A double value.
- See Also:
- nextDouble
getInt
public static int getInt(int hi)
- Generate a random number using the default generator.
- See Also:
- nextInt
getInt
public static int getInt(int lo,
int hi)
- Generate a random number using the default generator.
- See Also:
- nextInt
getLong
public static long getLong(long hi)
- Generate a random number using the default generator.
- See Also:
- nextLong
getLong
public static long getLong(long lo,
long hi)
- Generate a random number using the default generator.
- See Also:
- nextLong
getFloat
public static float getFloat(float hi)
- Generate a random number using the default generator.
- See Also:
- nextFloat
getFloat
public static float getFloat(float lo,
float hi)
- Generate a random number using the default generator.
- See Also:
- nextFloat
getDouble
public static double getDouble(double hi)
- Generate a random number using the default generator.
- See Also:
- nextDouble
getDouble
public static double getDouble(double lo,
double hi)
- Generate a random number using the default generator.
- See Also:
- nextDouble
All Packages Class Hierarchy This Package Previous Next Index