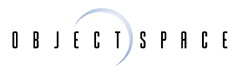
| JGL - The Generic Collection Library for Java |
All Packages Class Hierarchy This Package Previous Next Index
Class com.objectspace.jgl.adapters.IntArray
java.lang.Object
|
+----com.objectspace.jgl.adapters.ArrayAdapter
|
+----com.objectspace.jgl.adapters.IntArray
- public class IntArray
- extends ArrayAdapter
IntArray allows a native array of ints to be accessed like a JGL
Container. It is particularly useful for applying generic algorithms
such as Sorting.sort() to a native array.
-
IntArray()
- Construct myself to refer to an empty array.
-
IntArray(int[])
- Construct myself to refer to a native Java array.
-
IntArray(IntArray)
- Construct myself to refer to an existing IntArray.
-
IntArray(IntBuffer)
- Construct myself to be a copy of an existing IntBuffer.
-
at(int)
- Return the integer at the specified index as a Int object.
-
begin()
- Return an iterator positioned at my first item.
-
clone()
- Return a shallow copy of myself.
-
elements()
- Return an Enumeration of my components.
-
end()
- Return an iterator positioned immediately after my last item.
-
equals(int[])
- Return true if I contain the same items in the same order as
a native array of ints.
-
equals(IntArray)
- Return true if I contain the same items in the same order as
another IntArray.
-
equals(IntBuffer)
- Return true if I contain the same items in the same order as
another IntBuffer.
-
equals(Object)
- Return true if I'm equal to a specified object.
-
finish()
- Return an iterator positioned immediately after my last item.
-
get()
- Retrieve the underlying primitive array.
-
intAt(int)
- Return the integer at the specified index as a Int object.
-
maxSize()
- Return the maximum number of objects that I can contain.
-
put(int, int)
- Set the value of a specified index.
-
put(int, Object)
- Set the object at a specified index.
-
size()
- Return the number of objects that I contain.
-
start()
- Return an iterator positioned at my first item.
-
toString()
- Return a string that describes me.
IntArray
public IntArray()
- Construct myself to refer to an empty array.
IntArray
public IntArray(IntArray array)
- Construct myself to refer to an existing IntArray.
- Parameters:
- array - The IntArray to copy.
IntArray
public IntArray(IntBuffer buffer)
- Construct myself to be a copy of an existing IntBuffer.
- Parameters:
- array - The IntBuffer to copy.
IntArray
public IntArray(int array[])
- Construct myself to refer to a native Java array.
- Parameters:
- array - The int[] to ape.
clone
public synchronized Object clone()
- Return a shallow copy of myself.
- Overrides:
- clone in class ArrayAdapter
toString
public synchronized String toString()
- Return a string that describes me.
- Overrides:
- toString in class Object
equals
public boolean equals(Object object)
- Return true if I'm equal to a specified object.
- Parameters:
- object - The object to compare myself against.
- Returns:
- true if I'm equal to the specified object.
- Overrides:
- equals in class ArrayAdapter
equals
public boolean equals(IntArray object)
- Return true if I contain the same items in the same order as
another IntArray.
- Parameters:
- array - The IntArray to compare myself against.
- Returns:
- true if I'm equal to the specified object.
equals
public boolean equals(IntBuffer buffer)
- Return true if I contain the same items in the same order as
another IntBuffer.
- Parameters:
- buffer - The IntBuffer to compare myself against.
equals
public synchronized boolean equals(int array[])
- Return true if I contain the same items in the same order as
a native array of ints.
- Parameters:
- array - The array to compare myself against.
get
public int[] get()
- Retrieve the underlying primitive array.
size
public int size()
- Return the number of objects that I contain.
maxSize
public int maxSize()
- Return the maximum number of objects that I can contain.
- Overrides:
- maxSize in class ArrayAdapter
elements
public Enumeration elements()
- Return an Enumeration of my components.
start
public ForwardIterator start()
- Return an iterator positioned at my first item.
begin
public synchronized IntIterator begin()
- Return an iterator positioned at my first item.
finish
public ForwardIterator finish()
- Return an iterator positioned immediately after my last item.
end
public synchronized IntIterator end()
- Return an iterator positioned immediately after my last item.
at
public Object at(int index)
- Return the integer at the specified index as a Int object.
- Parameters:
- index - The index.
intAt
public synchronized int intAt(int index)
- Return the integer at the specified index as a Int object.
- Parameters:
- index - The index.
put
public void put(int index,
Object object)
- Set the object at a specified index. The object must be a Number
- Parameters:
- index - The index.
- object - The object to place at the specified index.
- Throws: ClassCastException
- if object is not a Number
- Throws: IndexOutOfBoundsException
- if index is invalid.
put
public synchronized void put(int index,
int object)
- Set the value of a specified index.
- Parameters:
- index - The index.
- object - The int to place at the specified index.
- Throws: IndexOutOfBoundsException
- if index is invalid.
All Packages Class Hierarchy This Package Previous Next Index